Consuming an API with Postman
A hands-on tutorial that demonstrates the first steps for setting up a collection and consuming a simple API in Postman.
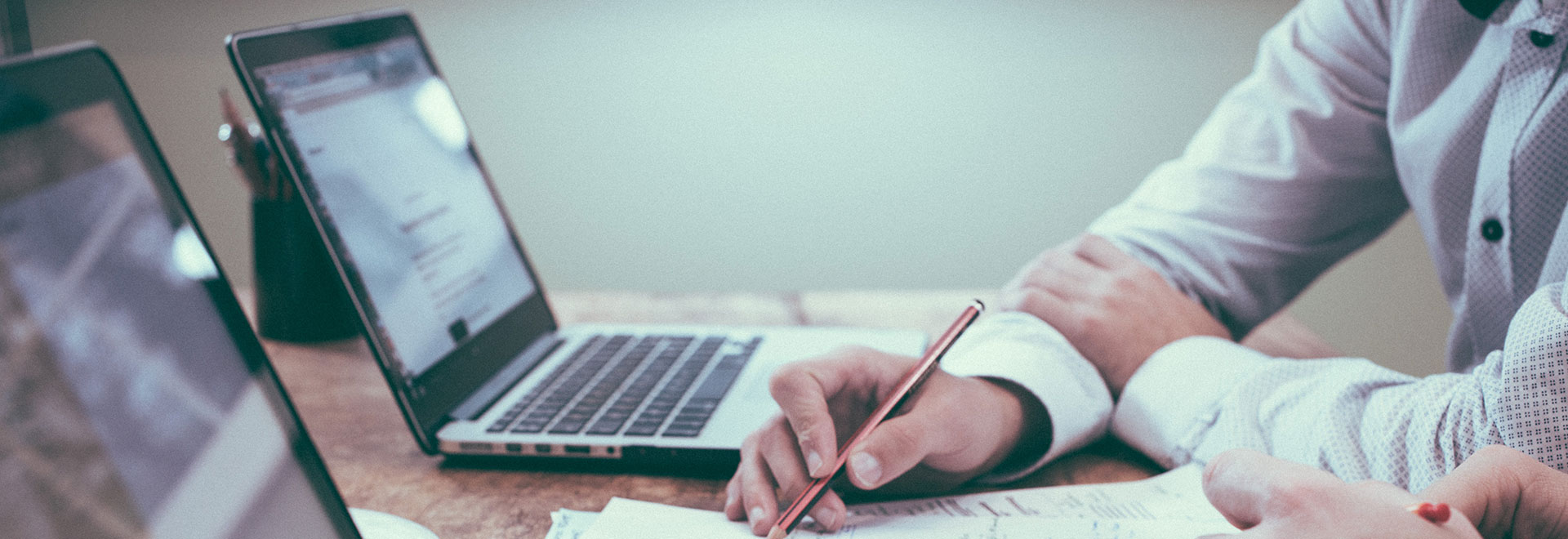
This tutorial is for you if you want to learn how to access an API with Postman.
Postman is a desktop application that allows you to craft HTTP requests such as API calls in a visual user interface and store and organize different requests in collections for reuse. It comes with a huge set of additional features to assist with API development, but this article only touches on the basics.
We have chosen Postman for the tutorial because it is widely used by millions of developers around the world and thus can be considered a standard. All functionality shown in this tutorial is available without registration and in the free version.
The API
BASF provides the Periodic Table of Elements API, which enables you to query the periodic table and retrieve all kinds of information about chemical elements.
Understanding the API
We assume you came here from the Introduction to APIs. If not, please check out that document to learn the basics of APIs before continuing.
The Periodic Table of Elements API follows the CRUD/RESTful paradigm but implements only a subset. Specifically, the periodic table is read-only and allows HTTP GET requests exclusively. It is also limited to one resource called "element."
For this resource, there are two endpoints. The first is a collection endpoint that allows you to either retrieve the full periodic table or request a subset of elements through filter parameters. It is called a collection endpoint because it always returns a collection data structure containing multiple items. The second is an individual resource endpoint that allows you to retrieve a single element.
We will discuss the URL structure for the endpoints later in this tutorial as we're building a collection containing both.
Prerequisites for Use
All BASF APIs require authentication. The Periodic Table of Elements API uses an app-only context with an API key. If you haven't done so, please read the introduction, terminology, and app-only context sections of the Authentication and Authorization guide. You can skip the part on the app and user context and OAuth as it is not required for this API.
To use authentication, you need to create an account and an application. The process gives you a Client ID, which you can then use as your API key. If you do not have an API key, follow the steps outlined in the Get Started guide. Make sure you select the API product BASF Periodic Table while creating your app.
Running Postman
You can download Postman for free from the official website, install it on your computer, and start it.
When you open the application, you can skip any welcome or registration screens and head straight to the main window.
Writing your First Request
As our first exercise, we'll send a request to the API directly from the Postman interface without creating any abstractions or collections.
In the right section of the Postman window, click on the plus icon ( + ) to open a new tab with an empty request.
Enter the URL https://dev.api.basf.com/chemical/elements/He in the field labeled "Enter request URL". That URL points to the individual resource endpoint for Helium (He). You can choose the HTTP verb with the dropdown in front of the URL field, though it should default to GET already.
The Periodic Table of Elements API allows you to provide your API key as the query parameter called x-api-key. Query parameters are the (typically) final part of the URL, starting with a question mark (?). You could add the parameter directly in the URL field, though it's easier to use the dedicated "Query Params" section. Enter x-api-key in the Key column and your Client ID from the BASF developer portal in the Value column.
Press the Send button. In an instant, you'll receive the API response below:
{
"number": 2,
"symbol": "He",
"group": "18",
"period": 1,
"name": "helium",
"atomic_mass": 4.002602,
"covalent_radius": 0.93,
"atomic_volume": 19.5,
"cas_registry_no": "7440-59-7",
"electrical_conductivity": null,
"specific_heat": 5.193,
"heat_of_fusion": null,
"heat_of_vaporization": 0.0845,
"thermal_conductivity": 0.152,
"polarizability": 0.198,
"heat_atomization": 0,
"year_discovered": 1895
}
What you see here is a JSON structure for a single element – in this case, Helium – with multiple attributes describing the element.
Creating a Collection
Now that we've confirmed our initial request worked, let's see how we can use a Postman collection to represent the API and understand its structure in the process.
Setting up the Basics
Click the New button and choose Collection. Give a name to your collection, e.g., "Periodic Table API".
In the previous section you learned that this API uses a query parameter for authentication. Since authentication is required for all the endpoints, it is useful to define it in the collection instead of individual requests. Head to the Authorization tab and choose API Key as the type. Enter x-api-key in Key and your Client ID in Value and choose Query Params under Add to.
Postman allows you to declare variables which can contain arbitrary values that you can reuse within the collection. We can create a variable to reference the base URL. That is the URL that every endpoint starts with, and in the BASF Periodic Table of Elements API it is https://dev.api.basf.com/chemical/. Click on the Variables tab and enter a new row by typing baseUrl in the Variable field and setting the Initial Value to https://dev.api.basf.com/chemical (without a trailing slash). Postman automatically copies this URL to Current Value as well.
We have now completed the overall settings for our collections. Click the Create button to confirm and save your collection.
Requesting All Elements
In the Periodic Table of Elements API, as in most properly designed APIs following a CRUD/RESTful paradigm, the collection endpoint is the base URL followed by the pluralized name of the resource. It follows that https://dev.api.basf.com/chemical/elements is the collection endpoint for elements.
Click on the three dots ( … ) that appear when you hover over your collection name in the list on the left side of the Postman window and choose Add Request from the menu.
Enter a name for your request, such as "All Elements", and confirm with the Save to (Collection Name) button.
Select the request you just created (you may have to expand your collection) and Postman opens it in a new tab. Now you can provide the URL. As you've already stored the base URL in a variable you can reuse it here. Type {{baseUrl}}/elements in the "Enter request URL" field. The double curly brackets indicate a reference to a collection variable.
Click Send to test your request. You should get a JSON structure with an items attribute containing multiple elements.
After you've confirmed it works, click Save to update your collection.
Adding Pagination
The periodic table contains 118 elements. In other APIs, you may have hundreds or thousands of instances of a specific resource. Getting them all in a single API call may put a strain both on the API server as well as your consuming application. To prevent that, APIs typically support pagination.
As a user, you may have seen pagination in search engine results where you were presented with the first ten results and then had to move on to the next page to see upcoming results.
BASF APIs, including the Periodic Table, use a pagination pattern based on offset and limit query parameters. With a limit, you can tell the API how many items you want to receive at once. For example, if you set the limit to 5, you only receive five results. Offset, on the other hand, tells the system to skip the first results. Assuming you already retrieved 5 results, you can set the offset and the limit to 5 to skip the first five items and retrieve the next five.
Let's try this in Postman. Under "Query Params", add a parameter by setting the key to offset and value to 5. Another line appears. Add the second parameter by setting the key to limit and value to 5. Click Send to try another API call and confirm that the response contains only five entries under items.
Postman shows a checkbox in front of every query parameter, enabling you to quickly test requests with or without them. You can therefore add all parameters that your endpoint allows and keep them disabled while saving your request. Next time you use your stored request you can identify and enable those parameters you need.
Adding Filters
Another common requirement for querying an API is to apply a filter to return only a subset of the resources. You can use a filter by adding a query parameter with the same name as the attribute by which you want to filter. The BASF Periodic Table of Elements API supports two filter parameters, group and period.
You can test the filter, for example by adding the key period and the value 6 to see all elements that appear in the sixth period of the periodic table.
Setting the Language
Other parameters can modify the way results are displayed. In the BASF Periodic Table of Elements API, results have a name attribute which contains the human-readable name of the respective chemical element. However, as humans use different languages, the API provides a language parameter through which you can make a choice. The API defaults to English if the parameter is not present and you can set the value of the parameter to an ISO language code, for example, de for German.
Sorting Results
The final parameter for the collection endpoint that we look at is the sort parameter. You can use it to change the order of the results. The value of this parameter is the attribute that you want to sort by, prefixed by either + for ascending results or - for descending results. Again, this is a pattern you can see in various BASF APIs.
Let's look at an example: if you want to retrieve elements in the order in which they were discovered, starting from the oldest, you can add the query parameter sort with value set to +year_discovered.
Requesting a Single Element
In the previous sections we have looked at all the ways in which you can tweak the results from the collection endpoint. Now it's time to add a second request to our collection, the endpoint for a single element.
The endpoint URL for a single resource is the related collection endpoint, followed by a slash and the identifier of the resource. It follows that https://dev.api.basf.com/chemical/elements/:id is the resource endpoint for a single element.
We have already used this endpoint during our first test request. What you've seen above at the end of the URL with the colon prefix is a placeholder. In the OpenAPI standard, placeholders for path parameters are commonly written with curly brackets, but Postman uses this alternate syntax.
Once more, click on the three dots ( … ) that appear when you hover over your collection name in the list on the left side of the Postman window and choose Add Request from the menu. Enter a name for your request, such as "Single Element", and confirm with the Save to (Collection Name) button.
Open the request you just created. Now you can provide the URL, again, reusing your base URL variable. Type {{baseUrl}}/elements/:id in the "Enter request URL" field. Notice that as soon as you enter :id, a new section "Path Variables" appears below "Query Params". The id can be either the number or the symbol of the element.
You can try out retrieving an element by entering a symbol like C in the value column for the id path variable. Click Send and you should see the details for carbon.
Make sure to Save your request. You've got a complete collection with two stored requests now!
Final Remarks
Congratulations on making it through the tutorial! We hope it deepened your understanding of APIs as well as the Postman application. Feel free to contact developer@basf.com with feedback and questions.